Calculate Business Days using LINQ
In this blog post, I will show one simple way to get the total business days within given range of two dates. Question related to this topic was asked several times in many ASP.NET / .NET Communities, so I thought it would be very good to document it with a blog post.
Since LINQ provides great ways to solve such requirements, in the following code I’m using LINQ’s functions.
First of all, I’ve created BusinessDays class (you can name it as you want) which contains two methods:
- WorkingDay, returns true/false. True if date is not Saturday or Sunday.
- GetAlldates, accepts two DateTime parameters where the first is the start date and the second is the end date of the Date range within we want to calculate the number of business days.
{
public static bool WorkingDay(this DateTime date)
{
return date.DayOfWeek != DayOfWeek.Saturday && date.DayOfWeek != DayOfWeek.Sunday;
}
/* this function is used only to collect the dates
we are not paying much attention of the implementation */
public static List<DateTime> GetAlldates(DateTime start, DateTime end)
{
List<DateTime> dates = new List<DateTime>();
DateTime currLoopDate = start;
while (currLoopDate < end)
{
dates.Add(currLoopDate);
currLoopDate = currLoopDate.AddDays(1);
}
return dates;
}
}
Once we’ve created this class, here is how to implement it within your web application:
I’ve used the Button1_Click method which is triggered by the Button’s Click event. I only need to call two lines of code.
int getBizDays = dates.Where(day => day.WorkingDay()).Count();
#1 using BusinessDays.GetAlldates(DateTime.Now, DateTime.Now.AddDays(7)); I’m calling the GetAllDates static method from the BusinessDays static class, so that it will retrieve me a List of Dates within the given start-end range.
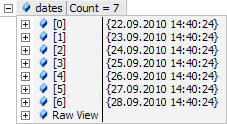
#2 in getBizDays I get the number of days calculated using the LINQ functions Where and Count.
EDITED
RichardD suggested even better implementation of the GetAlldates method using IEnumerable
{
DateTime currLoopDate = start;
while (currLoopDate < end)
{
yield return currLoopDate;
currLoopDate = currLoopDate.AddDays(1);
}
}
Also, we can shorten the dates.Where(day => day.WorkingDay()).Count() to only dates.Count(day => day.WorkingDay()).
Thanks RichardD
----
Hope this was useful post.
Regards,
Hajan